Routing
Simple, file-based routing to RESTful Zig function declarations.
HTML
Templating with Zmpl provides layouts, partials, and build-time static content rendering.
JSON
All endpoints render JSON by default, providing a RESTful API for free.
Speed
Powered by http.zig for competitive performance and scalability.
Tooling
User-friendly CLI tooling for creating projects and adding new components.
Middleware
Hook into and manipulate requests/responses with a custom middleware chain. Built-in middleware for htmx .
Cookies
Cookies, user sessions, and request/response headers out of the box.
Community
Join us on Discord – we're friendly and active.
Open Source
Free, open source, and always will be. Jetzig is MIT licensed.
Simple, concise view functions
const std = @import("std");
const jetzig = @import("jetzig");
pub const layout = "application";
pub fn index(request: *jetzig.Request, data: *jetzig.Data) !jetzig.View {
var object = try data.object();
try object.put("url", data.string("https://jetzig.dev/"));
try object.put("title", data.string("Jetzig Website"));
try object.put("message", data.string("Welcome to Jetzig!"));
return request.render(.ok);
}
Modal templating with partials, Markdown, and Zig
<html>
<body>
@partial header
@markdown {
# {{.message}}
[{{.title}}]({{.url}})
}
@zig {
if (10 > 1) {
<span>10 is greater than 1!</span>
}
}
<p>{{.message}}</p>
@partial link(href: .url, title: .title)
@partial footer
</body>
</html>
JSON by default on all endpoints
$ curl http://localhost:8080/example.json
{
"url": "https://jetzig.dev/",
"message": "Welcome to Jetzig!",
"title": "Jetzig Website"
}
Readable development logs
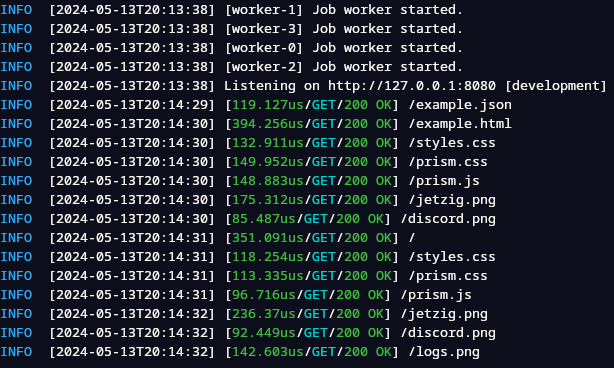
Easy email delivery with content templating
pub fn post(request: *jetzig.Request, data: *jetzig.Data) !jetzig.View {
var object = try data.object();
const params = try request.params();
// Set mail template params.
try object.put("from", params.get("from"));
try object.put("message", params.get("message"));
// Create email.
const mail = request.mail("contact", .{ .to = &.{"hello@jetzig.dev"} });
// Deliver asynchronously.
try mail.deliver(.background, .{});
return request.render(.created);
}
Background jobs
pub fn index(request: *jetzig.Request, data: *jetzig.Data) !jetzig.View {
// Prepare a job using `src/app/jobs/example.zig`.
var job = try request.job("example");
// Add params to the job.
try job.params.put("foo", data.string("bar"));
try job.params.put("id", data.integer(std.crypto.random.int(u32)));
// Schedule the job for background processing.
try job.schedule();
return request.render(.ok);
}